Country, State, County, City, Zip, Neighborhood, Street, Unit, and Coordinates. That’s how we break down our location information for any property. This can be seen in the results from an API call for a listing as shown here:
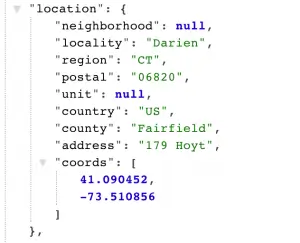
Sometimes that isn’t enough. Neighborhood in most cases is mapped to sub-division. But in some places the sub-division isn’t what people think of as a neighborhood, there might be something bigger than a subdivision but smaller than a city. Or other times there is a level smaller than a sub-division.
The challenge is in supporting these additional levels. What we have been doing so far is adding a new field to the uncur_data group of our feed. In most cases this is area which is a superset of neighborhoods (sub-divisions). And while it is useful to have this information in the feed, it isn’t always convenient to display the information.
Recently a partner asked for a solution to this problem and they pointed me to the HiltonHeadMLS.com website for a potential solution. On that site, there is a dropdown for Area and sub-Areas which is simple and quite elegant. When you select an Area, you are shown all the properties for that area. Select a sub-Area and the list is filtered down accordingly.
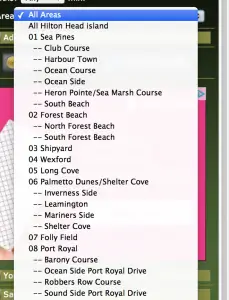
So how could we provide a dropdown like this? Well it’s definitely possible with shortcodes, but not exactly obvious how. One option would be to generate the information at runtime. Thats actually what we do for every dropdown on the site. We can call the aggregate function off of listing and pass it a set of keys to aggregate on along with a filter to see a list of the options available. So I could set the filter to Area 1, which in the case of Hilton Head, is Sea Pines then aggregate sub-Area to see the list including Club Course, Harbor Town, Ocean Course, Ocean Side, Heron Pointe, and South Beach. The rest-ful call to do that is:
https://api.placester.com/v2.1/listings/aggregate?api_key=YourAPIKeyHere&location[neighborhood]=Sea%20Pines&keys[]=uncur_data.sub_area
The benefit of this is that it is always the freshest information. The downside is that although we target a super quick response time for our API calls, one for each of 40+ neighborhoods still adds up to some time approaching a full second.
I decided it would be much better to provide a static JSON object which represented the neighborhoods and sub-areas which I could iterate through. The only cost here is getting the list of areas converted to a JSON object. With a little text wizardy in SublimeText3 and Keyboard Maestro, I was able to convert the source for the dropdown on the HiltonHeadMLS site to what I needed.
To convert that object into a set of options in an HTML select, I used just a touch of javascript:
for (hood in neighborhoods) {
jQuery(“#hoodselect”).append(buildOptions(hood,neighborhoods[hood]));
}
function buildOptions(parent, childArray){
output= createOption(parent,parent);
childArray.forEach(function(child){
output+=createOption(‘– ‘+ child, child);
});
return output;
};
function createOption(key,value){
return ‘<option value=”‘ + value +'”>’ + key + ‘</option>’;
};
This basically iterates through my neighborhoods then builds out options for the neighborhood name and then each sub area in the array, inserting a double dash for each sub area.
Now that I have a dropdown with all of my areas and subareas, I need to get the results to help build the right query to run. If the selected item is a neighborhood, then I need to do a location[neighborhood]=selectedNeighborhood. If it isn’t a neighborhood, then I need to do metadata[sub_area]=selectedSubArea. That is achieved in the following code:
jQuery(“#hoodselect”).change(function () {
str = jQuery(“#hoodselect”).val();
if(neighborhoods.hasOwnProperty(str)){
jQuery(“#location-neighborhood”).val(str);
jQuery(“#metadata-sub_area”).val(“”);
} else {
jQuery(“#location-neighborhood”).val(“”);
jQuery(“#metadata-sub_area”).val(str);
}
})
In the HTML for the Shortcode Template I have a dropdown with an id of hoodselect and two hidden textboxes with id’s location-neighborhood and metadata-sub_area. These have names of location[neighborhood] and metadata[sub_area] respectively. The names are what lets the form perform the appropriate query.
If you want to see the whole thing put together in a Gist, check it out here: https://gist.github.com/technovangelist/8591395
The two gotchas I ran into here were needing to spell out jQuery instead of the $ shortcode and having to escape at least one of the brackets for neighborhood in the HTML block. I have run into those before but I always forget and blow away far too much time.
When you are done, you should get a dropdown that looks something like this:
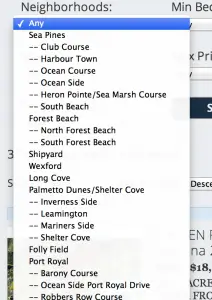
Of course, in your environment you will have to adjust to whatever the fields are for your MLS.
I hope this article is useful for you and that you can see how powerful and flexible Placester Shortcodes can be. If you have any questions, please let us know.